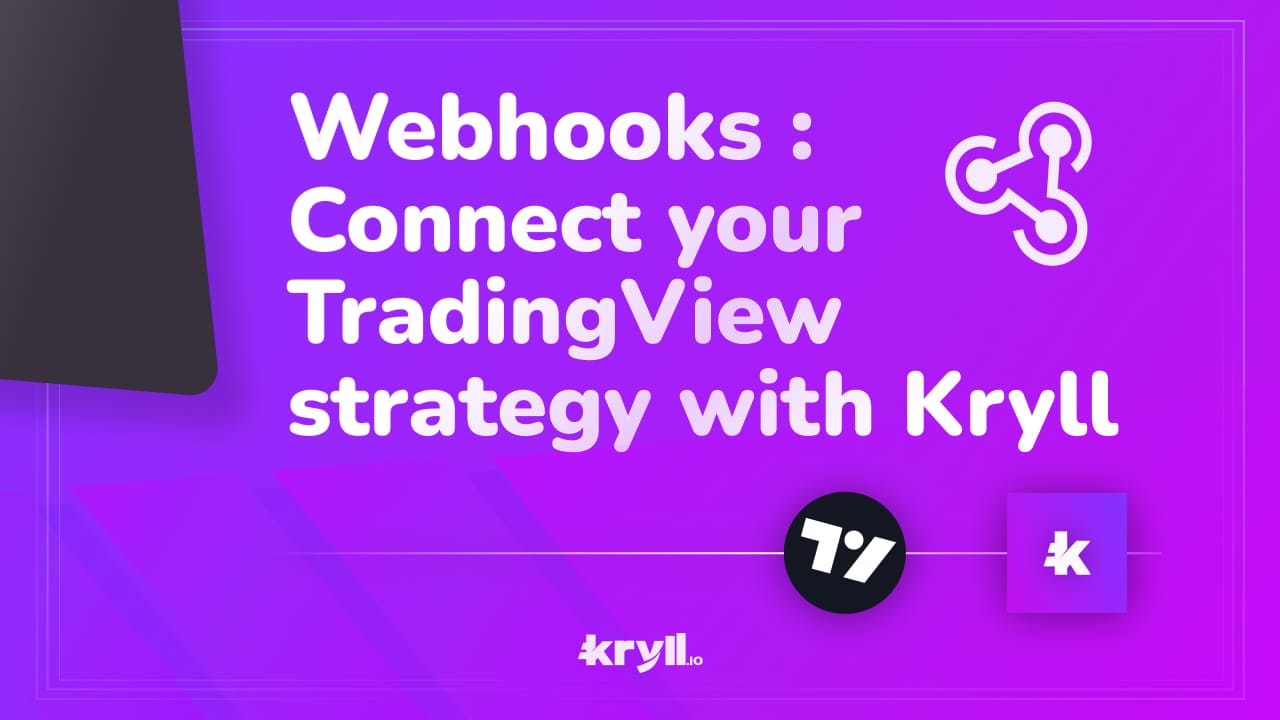
With the arrival of Webhooks blocks on Kryll, users can now drive their strategies usign an external or personalized platform. In this article, we show you how to run a Kryll strategy algorithm via PineScript (a programming language developed by TradingView for strategy and indicator creation).
Prerequisite: You must have at least a Pro account on TradingView to be able to generate Webhook alerts.
Creating a TradingView strategy
We will use a TradingView example strategy developed in Pinescript v5.
In this example, the strategy will use two technical indicators that are not available on Kryll: the intersection of two moving averages of RSI and the Vortex indicator. Depending on the configuration of these indicators, the strategy will place Long, Short or Close orders.
//@version=5
// KRYLL Webhook - Tradingview strategy Exemple
strategy("Demo Strat", overlay=true)
// inputs zone
rsi_length = input.int(7, title="RSI Length", minval=2)
rsi_fma_length = input.int(7, title="Fast RSI MA Length", minval=2)
rsi_sma_length = input.int(14, title="Slow RSI MA Length", minval=4)
rsi_neutral = input.int(20, title="RSI neutral zone", minval=2)
vortex_period = input.int(14, title="Vortex Length", minval=2)
// Vortex indicator computation
VMP = math.sum( math.abs( high - low[1]), vortex_period )
VMM = math.sum( math.abs( low - high[1]), vortex_period )
STR = math.sum( ta.atr(1), vortex_period )
VIP = VMP / STR
VIM = VMM / STR
// Fast and Slow RSI Moving Average computation
rsi = ta.rsi(close, rsi_length)
rsiFMA = ta.sma(rsi, rsi_fma_length)
rsiSMA = ta.sma(rsi, rsi_sma_length)
// Fast and Slow RSI cross detection
co = ta.crossover(rsiFMA, rsiSMA)
cu = ta.crossunder(rsiFMA, rsiSMA)
if (not na(rsiSMA))
if (VIP < VIM)
if( cu )
strategy.entry("trade", strategy.short, comment="Short")
else
strategy.close(id="trade", comment="Close")
if (VIP > VIM)
if( co )
strategy.entry("trade", strategy.long, comment="Long")
else
strategy.close(id="trade", comment="Close")

Study of the Tradingview strategy
This strategy generates 3 types of orders depending on the configuration of the indicators used.
strategy.entry("trade", strategy.long, comment="Long")
strategy.entry("trade", strategy.short, comment="Short")
strategy.close(id="trade", comment="Close")
We will have to create a Kryll strategy that will wait for one of these 3 signals to execute the corresponding trade. In this TradingView strategy, we can see that a long or short position can be followed by their opposite without necessarily going through a close first. The strategy should be designed accordingly.

Implementation of the strategy in Kryll
The implementation of this Kryll strategy that will be driven via Webhooks could look like this.
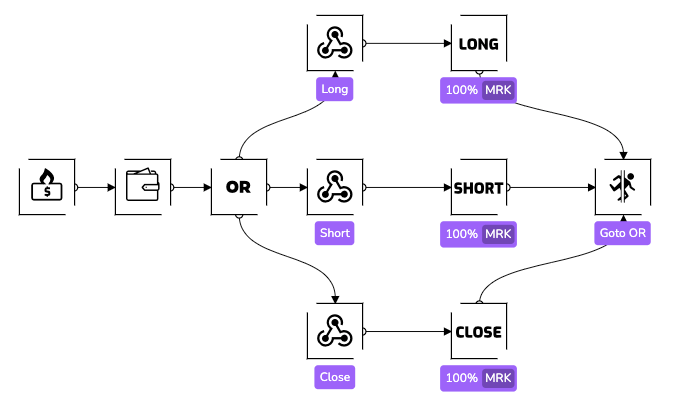
Each webhook block will provide you with a URL generic to the strategy and a Payload message specific to each block. This information must be provided to TradingView through the pinescript code.
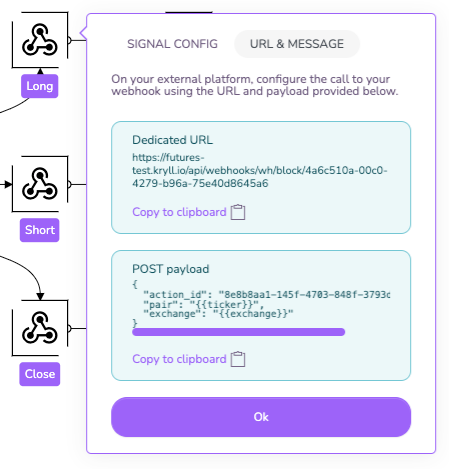
At this point our strategy is complete, we can even launch it.

Adaptation of the TradingView strategy
We will now move on to modifying your TradingView strategy to allow it to send signals to the Kryll strategy.
First of all, let's modify the beginning of the strategy to add some useful statements for the following:
//@version=5
// KRYLL Webhook - Tradingview strategy Exemple
strategy("Demo Strat", overlay=true)
// Kryll Webhook declarations
payload_start="{\"action_id\" : \""
payload_end="\",\"pair\":\""+syminfo.ticker+"\"}"
kryll_action=""
payload=""
Let's add the variables that will allow us to store and use the 'action_id' of our different Webhook blocks:
id_block_long = "put here your block id"
id_block_short = "put here your block id"
id_block_close = "put here your block id"
Finally, let's modify some position entry/exit/close calls (strategy.entry and strategy.close) so that the TradingView strategy can build and send the right message to Kryll.
if (not na(rsiSMA))
if (VIP < VIM)
if( cu )
payload := payload_start + id_block_short + payload_end
strategy.entry("trade", strategy.short, comment="Short", alert_message=payload)
else
payload := payload_start + id_block_close + payload_end
strategy.close(id="trade", comment="Close", alert_message=payload)
if (VIP > VIM)
if( co )
payload := payload_start + id_block_long + payload_end
strategy.entry("trade", strategy.long, comment="Long", alert_message=payload)
else
payload := payload_start + id_block_close + payload_end
strategy.close(id="trade", comment="Close", alert_message=payload)
Final Result
Here is what the finalized script looks like.
//@version=5
// KRYLL Webhook - Tradingview strategy Exemple
strategy("Demo Strat", overlay=true)
// Kryll Webhook declarations
payload_start="{\"action_id\" : \""
payload_end="\",\"pair\":\""+syminfo.ticker+"\"}"
kryll_action=""
payload=""
id_block_long = "8f94aaf9-0f37-4730-8565-a40c8f151921"
id_block_short = "8f94aaf9-2f30-7430-2364-519a40c8f121"
id_block_close = "8f94aaf9-1a37-3540-5431-c8f121519a40"
// inputs zone
rsi_length = input.int(7, title="RSI Length", minval=2)
rsi_fma_length = input.int(7, title="Fast RSI MA Length", minval=2)
rsi_sma_length = input.int(14, title="Slow RSI MA Length", minval=4)
rsi_neutral = input.int(20, title="RSI neutral zone", minval=2)
vortex_period = input.int(14, title="Vortex Length", minval=2)
// Vortex indicator computation
VMP = math.sum( math.abs( high - low[1]), vortex_period )
VMM = math.sum( math.abs( low - high[1]), vortex_period )
STR = math.sum( ta.atr(1), vortex_period )
VIP = VMP / STR
VIM = VMM / STR
// Fast and Slow RSI Moving Average computation
rsi = ta.rsi(close, rsi_length)
rsiFMA = ta.sma(rsi, rsi_fma_length)
rsiSMA = ta.sma(rsi, rsi_sma_length)
// Fast and Slow RSI cross detection
co = ta.crossover(rsiFMA, rsiSMA)
cu = ta.crossunder(rsiFMA, rsiSMA)
if (not na(rsiSMA))
if (VIP < VIM)
if( cu )
payload := payload_start + id_block_short + payload_end
strategy.entry("trade", strategy.short, comment="Short", alert_message=payload)
else
payload := payload_start + id_block_close + payload_end
strategy.close(id="trade", comment="Close", alert_message=payload)
if (VIP > VIM)
if( co )
payload := payload_start + id_block_long + payload_end
strategy.entry("trade", strategy.long, comment="Long", alert_message=payload)
else
payload := payload_start + id_block_close + payload_end
strategy.close(id="trade", comment="Close", alert_message=payload)

Creating an alert in Tradingview
Once you have finalized your script, set the pair and time unit on which you want to run your TradingView strategy. In our case, we'll set the pair to ETH/USDT and a time unit of 2 hours.
Then go to the "Pine Editor" to paste our strategy and save it.
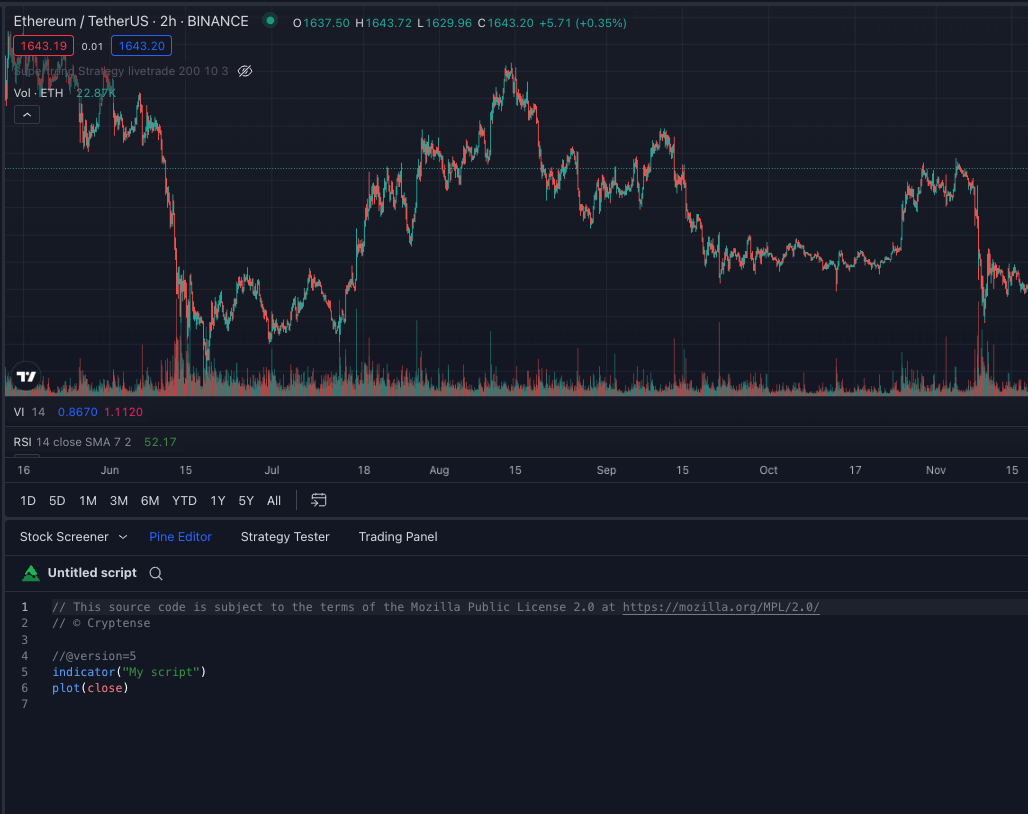
Let's add our strategy via the 'Indicators' button to make it appear on the chart.
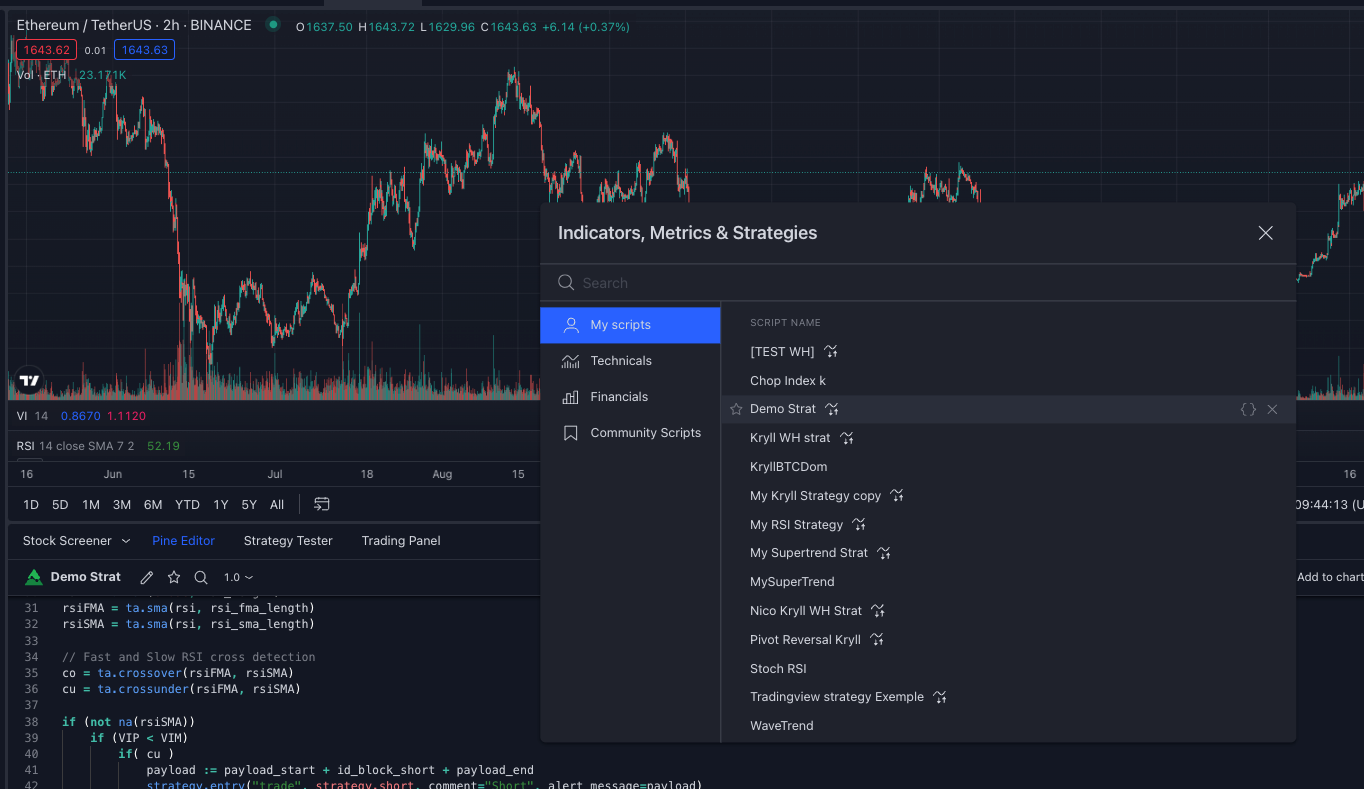
With our TradingView configured, let's now create the alert that will allow us to send messages to our strategy via webhook calls.
To do this, let's click on the 'Create Alert' button and configure it: select our strategy as a condition, name our alert and configure the message:
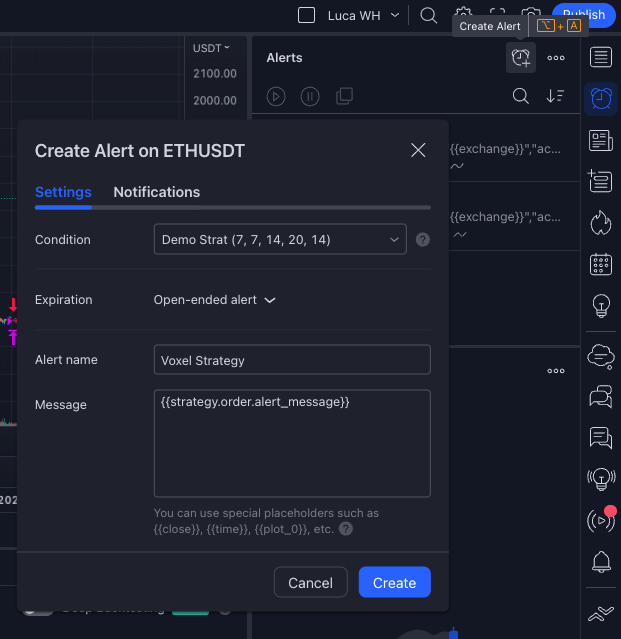
Next, let's set up the strategy's webhook URL. You will find this URL on one of the webhook blocks of your Kryll strategy.
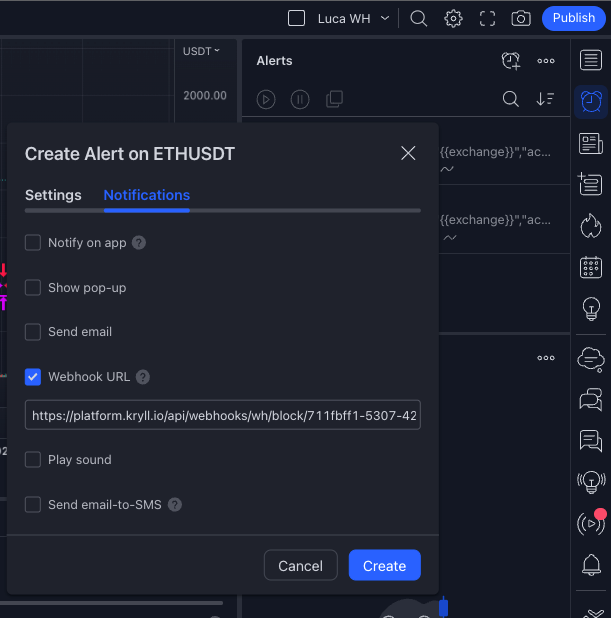
Finally, click on Create to validate the creation of your alert and you will be able to find your alert in the list of TradingView alerts.

Let TradingView and Kryll trade for you
At this point, if your alert is active on TradingView and your strategy is launched on Kryll, the two platforms should communicate.
To go further...
Enrich your creations
The example described in this tutorial remains simple and could be enriched by adding a Take Profit or a Stop Loss which would then be new Webhook blocks leading to another flow.
Create your own indicators
The interfacing of TradingView and Kryll opens up an infinite number of possibilities for Kryll users. You can now create your own technical indicators, mix them together, clone indicators or strategies from the TradingView community and use all of this to create your own automated trading strategies!
To help you with this task, here are two strategy templates that can serve as a starting point for your next Spot and Futures trades.
//@version=5
// KRYLL Webhook - Tradingview strategy template
strategy("Kryll Spot Template", overlay=true)
payload_start="{\"action_id\" : \""
payload_end="\",\"pair\":\""+syminfo.ticker+"\"}"
kryll_action=""
payload=""
kryll_action="none"
id_block_buy = "put here your block id"
id_block_sell = "put here your block id"
// ----------------------- YOUR CODE HERE ---------------
// [...]
// In your code, set kryll_action to 'buy' or 'sell' mode, depending on the desired behavior.
//
// exemple
// kryll_action := 'sell'
// ----------------------- KRYLL FINAL CODE ---------------
if (kryll_action == "buy")
payload := payload_start + id_block_buy + payload_end
strategy.entry("trade", strategy.long, comment="Buy", alert_message=payload)
if (kryll_action == "sell")
payload := payload_start + id_block_sell + payload_end
strategy.entry("trade", strategy.close, comment="Sell", alert_message=payload)
//@version=5
// KRYLL Webhook - Tradingview strategy template
strategy("Kryll Futures Template", overlay=true)
payload_start="{\"action_id\" : \""
payload_end="\",\"pair\":\""+syminfo.ticker+"\"}"
kryll_action=""
payload=""
kryll_action="none"
id_block_long = "put here your block id"
id_block_short = "put here your block id"
id_block_close = "put here your block id"
// ----------------------- YOUR CODE HERE ---------------
// [...]
// In your code, set kryll_action to 'long', 'short' or 'close' mode depending on the desired behavior.
//
// exemple
// kryll_action := 'sell'
// ----------------------- KRYLL FINAL CODE ---------------
if (kryll_action == "long")
payload := payload_start + id_block_long + payload_end
strategy.entry("trade", strategy.long, comment="Long", alert_message=payload)
if (kryll_action == "short")
payload := payload_start + id_block_short + payload_end
strategy.entry("trade", strategy.short, comment="Short", alert_message=payload)
if (kryll_action == "close")
payload := payload_start + id_block_close + payload_end
strategy.entry("trade", strategy.close, comment="Close", alert_message=payload)
Learn more
Various tutorials are available for more information. The Kryll team has several other articles related to this subject:
- Webhooks, the game-changer for Kryll trading algorithms
- The Webhook Block Guide
- Run your Kryll strategy bots with TradingView
Thank you for following this tutorial! To your Webhooks!

Happy Trading,
Website : https://kryll.io
Twitter : @Kryll.io
Telegram EN : https://t.me/kryll_io
Telegram FR: https://t.me/kryll_fr
Telegram ES: https://t.me/kryll_es
Discord : https://discord.gg/PDcHd8K
Facebook : https://www.facebook.com/kryll.io
Support : support@kryll.io